Introduction to Node.js and How Node.js works?
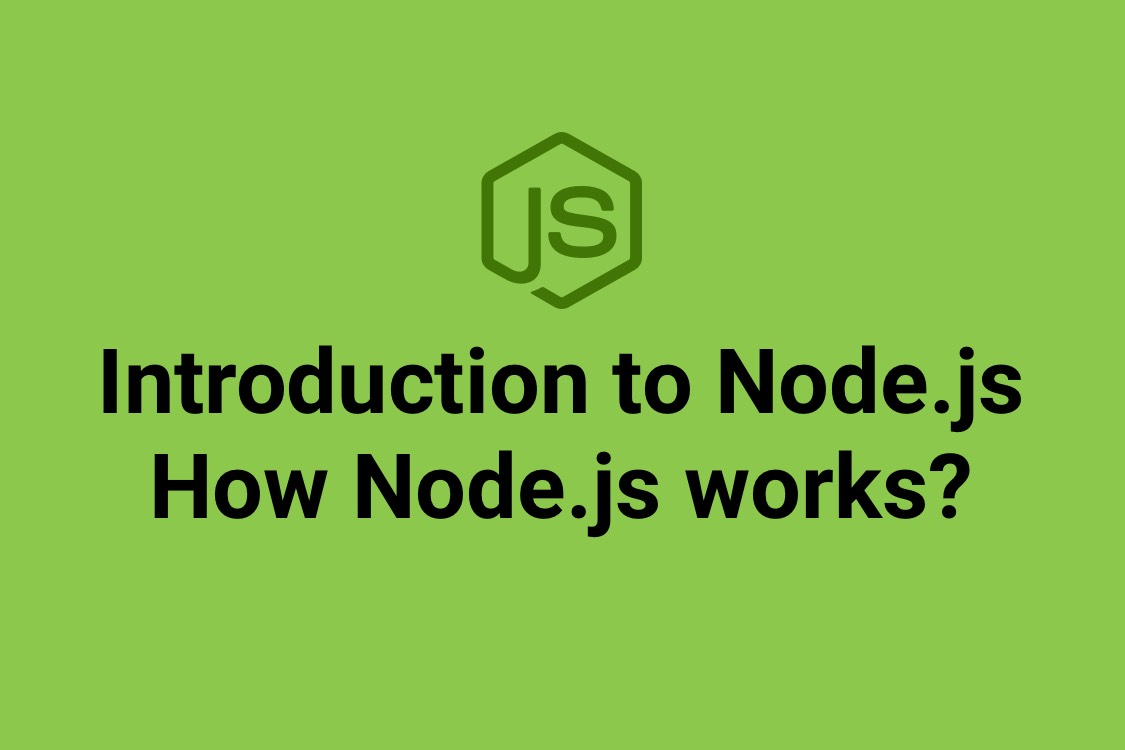
Node.js is a popular framework for building backend systems (network applications) which can scale very well. Node.js is a Javascript runtime i.e. it runs the javascript codes. Javascript has long been used in webpages and run by the browsers. But now the Javascript can also run without a browser. This was made possible via Node.js which uses Chrome's V8 javascript engine.
Now, you may be wondering how a scripting language i.e. Javascript can be used for developing performant applications. We do know that compiled codes are almost always much more efficient than the interpreted scripts. And to add to this argument, Javascript is a single-threaded language. So, how can Javascript be preferred over other alternatives for backend development? We will come back to this question in a while.
Javascript has greatly broadened its horizon and become much more than a browser scripting language. It is now being used in frameworks with radically different use-cases. Few use cases are as listed below.
- Websites - React.js, Angular, Vue.js, JQuery, and thousands more.
- Backend Systems - Node.js
- Persistence (Database) - Mongodb
- Mobile, and Desktop applications
Now coming back to the original question, how Node.js makes Javascript become performant comparatively?
To answer this question we need to get familiar with the below-mentioned concepts:
- Blocking and non-blocking operations.
- Node.js asynchronous callback mechanism.
- Node.js and V8 Javascript engine.
- Node.js event loop.
These topics will be covered in this blog series. There are a few more topics that I will also cover as listed below:
- Core modules of the Node.js.
- Introduction to Express.js.
Let's start with the understanding of a single-threaded system and concurrency in Node.js in this 1st part of the series.
Node.js is an asynchronous event-driven Javascript runtime. It is used to build scalable network applications.
Let's describe a few terms used in the above statement.
Asynchronous: It means that the different parts of a program can run simultaneously without blocking other parts of that program. Node.js is by design asynchronous. Many connections to the webserver can be handled concurrently and at each connection, a callback is fired.
What is the difference between concrurrency and asynchronous?
Concurrency: Multiple things happening at once.
Asynchronous: Ask for something to happen, get notified when it does. Do other stuff in the meantime.
Event-driven: It a software architecture in which a major change in some logic or properties also called State change is propagated within the application so that different concerning handlers can run based on this change. This change is called the Event. In Node.js each asynchronous operation like database connection is considered as an Event.
Javascript Runtime: It is like an environment or a secondary program running alongside your application that has everything needed to execute your javascript code. So, when you run a script in javascript, this environment is responsible to handle callstacks, memory allocation, and management, bytecode compilation and optimization. We will understand this in detail when we deal with the Chrome V8 engine.
Now let's take a look at a simple Node.js program.
Before you can create your own webserver, you need to install npm and node on your machine. There are many tutorials online to guide the installation based on the operating system on your machine.
Create a file and name it as server.js and paste the below code.
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});
server.listen(port, hostname, () => {
console.log(`Server listening at http://${hostname}:${port}/`);
});
When you run your web server using
, then you will get a log of the URL and the port server is listening. Visit node server.js
, and you will see a message 'Hello World'http://localhost:3000
In this example, http
is a core module provided by the Node.js for handling the web server connection. Here we have simply created a server and provided a callback function that will be fired whenever any client makes an API request. In the next block, we have specified the port and hostname for this server to listen to.
Simple enough! Now there are many interesting things governing behind the scenes for such a simple api to be made available for us by Node.js and also being great in performance. The aim of this article is to peel off this hidden layer and gain wisdom.
In the next article, we will look at the blocking and non-blocking operations, and how Node.js deals with asynchronous operations.
Link to the next article: Blocking and Non-Blocking in Node.js - Asynchronous Operations and Callbacks